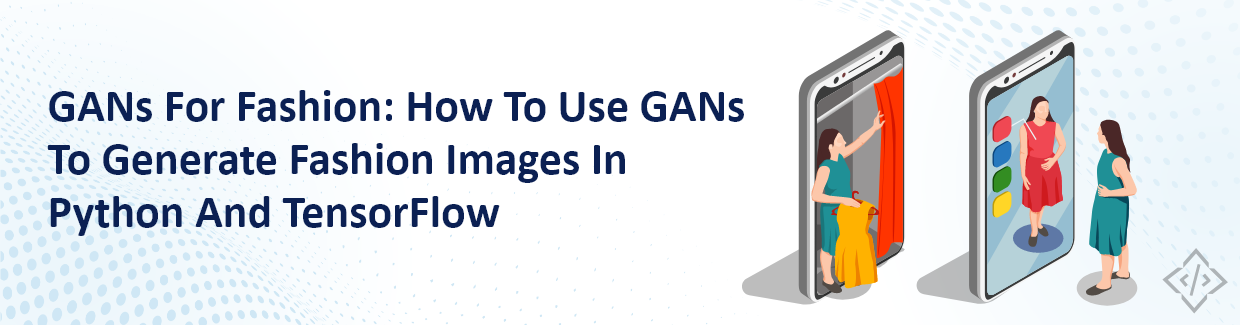
GANs For Fashion: How To Use GANs To Generate Fashion Images In Python And TensorFlow
In the ever-changing world of fashion, it is essential to stay ahead of the curve. Fashion designers, brands, and enthusiasts are always looking for innovative ways to push the boundaries of creativity. Generative Adversarial Networks (GANs) is a cutting-edge technologies that revolutionize the fashion industry.
Using GANs you can generate new and creative designs and fashion brands can experiment with new ideas and trends more quickly and efficiently. Also, used to create virtual fitting rooms and other interactive experiences to improve customer experience. In this blog post, we'll dive into the world of GANs and demonstrate how to create a GAN model to generate fashion images using Python and TensorFlow.
What are GANs?
GANs, short for Generative Adversarial Networks, class of machine learning models introduced by Ian Goodfellow and his colleagues in 2014. GANs have two neural networks: a generator and a discriminator. These networks are trained simultaneously in a fashion, where the generator tries to produce data that is indistinguishable from real data, while the discriminator tries to differentiate between real and fake data.
The generator's goal is to generate data samples that are so realistic that the discriminator can't tell them apart from real data. Over time, this process leads to the generation of highly realistic synthetic data.
Generate Fashion Images using GANs With Python and TensorFlow
Here's a step-by-step guide on how to use GANs to generate stunning fashion images With Python and TensorFlow.
Also Read: How To Train TensorFlow Object Detection In Google Colab: A Step-by-Step Guide
Step 1: Set Up Your Environment
Set up the GANs environment and load the Fashion MNIST dataset, which consists of grayscale images of fashion items like clothes and accessories.
#import necessary Python libraries import tensorflow as tf import tensorflow_datasets as tfds import matplotlib.pyplot as plt import numpy as np # Load the Fashion MNIST dataset ds = tfds.load('fashion_mnist', split='train') # Define a function to scale the images def scale_images(data): image = data['image'] return image / 255 # Preprocess the dataset ds = ds.map(scale_images) ds = ds.cache() ds = ds.shuffle(60000) ds = ds.batch(128) ds = ds.prefetch(64)
After the dataset has been preprocessed, it is ready to be used to train a deep learning model.
Step 2: Build the Generator and Discriminator
Next, we'll create the generator and discriminator neural networks. The generator will take random noise as input and produce synthetic fashion images, while the discriminator will evaluate the authenticity of the images.
from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Conv2D, Dense, Flatten, LeakyReLU, Reshape, Dropout, UpSampling2D # Define the generator model def build_generator(): model = Sequential() model.add(Dense(7*7*128, input_dim=128)) model.add(LeakyReLU(0.2)) model.add(Reshape((7,7,128))) model.add(UpSampling2D()) model.add(Conv2D(128, 5, padding='same')) model.add(LeakyReLU(0.2)) model.add(UpSampling2D()) model.add(Conv2D(128, 5, padding='same')) model.add(LeakyReLU(0.2)) model.add(Conv2D(128, 4, padding='same')) model.add(LeakyReLU(0.2)) model.add(Conv2D(128, 4, padding='same')) model.add(LeakyReLU(0.2)) model.add(Conv2D(1, 4, activation='sigmoid', padding='same')) return model # Create an instance of the generator test_generator = build_generator() # Define the discriminator model def build_discriminator(): model = Sequential() model.add(Conv2D(32, 5, input_shape=(28,28,1))) model.add(LeakyReLU(0.2)) model.add(Dropout(0.4)) model.add(Conv2D(64, 5)) model.add(LeakyReLU(0.2)) model.add(Dropout(0.4)) model.add(Conv2D(128, 5)) model.add(LeakyReLU(0.2)) model.add(Dropout(0.4)) model.add(Conv2D(256, 5)) model.add(LeakyReLU(0.2)) model.add(Dropout(0.4)) model.add(Flatten()) model.add(Dropout(0.4)) model.add(Dense(1, activation='sigmoid')) return model # Create an instance of the discriminator test_discriminator = build_discriminator()
Step 3: Compile the GAN
To compile a GAN, you need to specify the optimizers and loss functions for both the generator and discriminator. The optimizer is a training algorithm that helps the generator and discriminator learn. The loss function measures the performance of the generator and discriminator and is used to update their parameters.
from tensorflow.keras.models import Model from tensorflow.keras.optimizers import Adam from tensorflow.keras.losses import BinaryCrossentropy # Define optimizers and loss functions gen_opt = Adam(learning_rate=0.0001) descr_opt = Adam(learning_rate=0.00001) gen_loss = BinaryCrossentropy() descr_loss = BinaryCrossentropy() class FashionGAN(Model): def __init__(self, generator, discriminator, *args, **kwargs): # Pass through args and kwargs to base class super().__init__(*args, **kwargs) # Create attributes for gen and disc self.generator = generator self.discriminator = discriminator def compile(self, g_opt, d_opt, g_loss, d_loss, *args, **kwargs): # Compile with base class super().compile(*args, **kwargs) # Create attributes for losses and optimizers self.g_opt = g_opt self.d_opt = d_opt self.g_loss = g_loss self.d_loss = d_loss def train_step(self, batch): # Get the data real_images = batch fake_images = self.generator(tf.random.normal((128, 128, 1)), training=False) # Train the discriminator with tf.GradientTape() as d_tape: # Pass the real and fake images to the discriminator model yhat_real = self.discriminator(real_images, training=True) yhat_fake = self.discriminator(fake_images, training=True) yhat_realfake = tf.concat([yhat_real, yhat_fake], axis=0) # Create labels for real and fakes images y_realfake = tf.concat([tf.zeros_like(yhat_real), tf.ones_like(yhat_fake)], axis=0) # Add some noise to the TRUE outputs noise_real = 0.15*tf.random.uniform(tf.shape(yhat_real)) noise_fake = -0.15*tf.random.uniform(tf.shape(yhat_fake)) y_realfake += tf.concat([noise_real, noise_fake], axis=0) # Calculate loss - BINARYCROSS total_d_loss = self.d_loss(y_realfake, yhat_realfake) # Apply backpropagation - nn learn dgrad = d_tape.gradient(total_d_loss, self.discriminator.trainable_variables) self.d_opt.apply_gradients(zip(dgrad, self.discriminator.trainable_variables)) # Train the generator with tf.GradientTape() as g_tape: # Generate some new images gen_images = self.generator(tf.random.normal((128,128,1)), training=True) # Create the predicted labels predicted_labels = self.discriminator(gen_images, training=False) # Calculate loss - the trick to training to fake out the discriminator total_g_loss = self.g_loss(tf.zeros_like(predicted_labels), predicted_labels) # Apply backprop ggrad = g_tape.gradient(total_g_loss, self.generator.trainable_variables) self.g_opt.apply_gradients(zip(ggrad, self.generator.trainable_variables)) return {"d_loss":total_d_loss, "g_loss":total_g_loss} # Create an instance of the GAN fashion_gan = FashionGAN(test_generator, test_discriminator) fashion_gan.compile(gen_opt, descr_opt, gen_loss, descr_loss)
Once the GAN has been compiled, you can start training it on your data.
Step 4: Train the GAN
To train the GAN, you can use the following steps:
- Sample a batch of real images from the Fashion MNIST dataset.
- Sample a batch of random noise vectors.
- Generate synthetic fashion images from the random noise vectors using the generator.
- Train the discriminator to distinguish between real and synthetic fashion images.
- Train the generator to generate images that fool the discriminator.
Repeat steps 3-5 for a fixed number of epochs, or until the generator and discriminator have converged. Here is an example of how to train a GAN in TensorFlow:
# Define a directory to save generated images import os from tensorflow.keras.preprocessing.image import array_to_img from tensorflow.keras.callbacks import Callback os.mkdir('images') # Custom callback to generate and save images during training class ModelMonitor(Callback): def __init__(self, num_img=3, latent_dim=128): self.num_img = num_img self.latent_dim = latent_dim def on_epoch_end(self, epoch, logs=None): random_latent_vectors = tf.random.uniform((self.num_img, self.latent_dim,1)) generated_images = self.model.generator(random_latent_vectors) generated_images *= 255 generated_images.numpy() for i in range(self.num_img): img = array_to_img(generated_images[i]) img.save(os.path.join('images', f'generated_img_{epoch}_{i}.png')) # Train the GAN hist = fashion_gan.fit(ds, epochs=2000, callbacks=[ModelMonitor()])
Step 5: Save the Generator and Discriminator Models
Once training is complete, you can save the generator and discriminator models so that you can use them to generate new fashion images in the future. To save the generator and discriminator models in TensorFlow, you can use the following code:
# Save the generator model. test_generator.save('generator.h5') # Save the discriminator model. test_discriminator.save('discriminator.h5') Once the models have been saved, you can load them again and use them to generate new fashion images using the following code: import tensorflow as tf # Load the generator model. generator = tf.keras.models.load_model('generator.h5') # Load the discriminator model. discriminator = tf.keras.models.load_model('discriminator.h5') # Generate a new fashion image. noise = tf.random.normal([1, noise_dim]) synthetic_image = generator(noise) # Display the generated image. plt.imshow(synthetic_image[0]) plt.show()
This is just a basic example of how to save and load GAN models in TensorFlow. You can use this code to fit your specific needs.
Conclusion
In this blog post, we've explored the concept of GANs and demonstrated how to build a GAN model for generating fashion images using Python and TensorFlow. GANs have the potential to generate highly realistic synthetic data, and they find applications in various domains, including image generation, style transfer, and data augmentation. Feel free to experiment with different datasets and architectures to create your own GAN-based projects!
Do you need help with your AI or ML project? Contact CodeTrade India, a leading AI and ML development company in India. Hire AI and ML developers from CodeTrade to meet your specific needs. Contact CodeTrade today and get a free consultation!