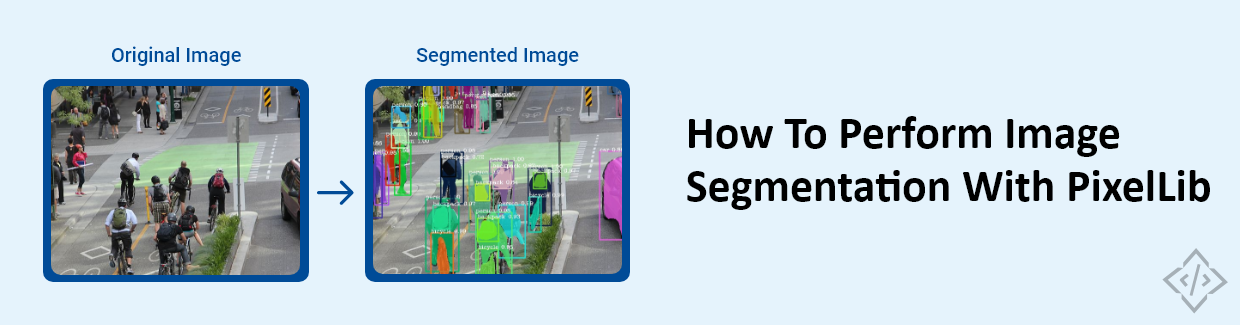
How To Perform Image Segmentation With PixelLib
Image segmentation is a computer vision technique that involves dividing an image into multiple segments or regions to identify and isolate objects or regions of interest. It is a powerful tool for a variety of tasks, such as object detection, image classification, and image editing. In this blog, we will explore how to perform image segmentation using Pixellib, step by step, and even extend it to video segmentation.
Overview of PixelLib
PixelLib is a Python library for image segmentation and object detection. It is a high-level wrapper around popular deep learning frameworks, such as TensorFlow and PyTorch, making it easy to perform image segmentation and object detection tasks with just a few lines of code.
In addition, PixelLib supports both semantic segmentation and instance segmentation. Semantic segmentation is the task of assigning each pixel in an image to a semantic class, such as "person", "car", or "tree". Instance segmentation is the task of assigning each pixel in an image to an instance of a semantic class, such as a specific person, car, or tree.
Step-by-Step Guide to Implement Image Segmentation Using PixelLib
Follow the given steps to perform image segmentation using PixelLib:
Step 1: Installation and Setup
Before we dive into image segmentation, you need to install the Pixellib library and download the pre-trained Mask R-CNN model. Open your Jupyter Notebook or any Python environment and run the following commands:
!pip install pixellib !wget --quiet https://github.com/matterport/Mask_RCNN/releases/download/v2.0/mask_rcnn_coco.h5
These commands will install Pixellib and download the Mask R-CNN model, which is pre-trained on the COCO dataset, a widely used benchmark for object detection and segmentation.
Step 2: Import Pixellib and Load the Model
Next, we'll import the necessary libraries, load the Mask R-CNN model, and configure Pixellib for instance segmentation. Here's the code for this step:
import pixellib from pixellib.instance import instance_segmentation segment_image = instance_segmentation(infer_speed="rapid") segment_image.load_model('mask_rcnn_coco.h5')
In this code snippet, we import Pixellib and initialize an instance_segmentation object. We also specify the inference speed as "rapid" for faster processing. After that, we load the pre-trained Mask R-CNN model.
Step 3: Perform Image Segmentation
Now, let's perform image segmentation on a sample image. Replace 'image.jpg' with the path to your own image. We will also display the bounding boxes around the detected objects and save the segmented image. Use the given code for implementation.
result, output = segment_image.segmentImage( 'image.jpg', show_bboxes=True, output_image_name='segmented_image.jpg' )
This code segment will segment the input image, display bounding boxes if specified, and save the segmented image as 'segmented_image.jpg'. The below image shows the original image view.
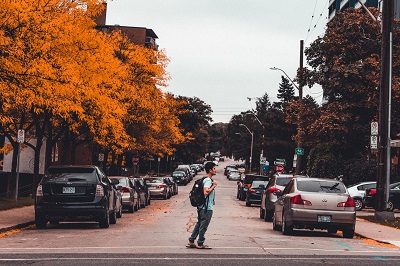
Step 4: Visualize the Segmented Image
To visualize the segmented image, you can use libraries like OpenCV and Matplotlib. Use the code to display the segmented image using Matplotlib:
import matplotlib.pyplot as plt import cv2 image = cv2.imread('segmented_image.jpg') plt.imshow(image) plt.show()
Output of the Image
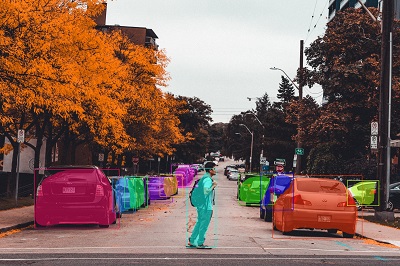
Step 5: Video Segmentation (Optional)
Also, Pixellib allows you to perform instance segmentation on videos. If you want to segment objects in a video, follow these steps.
import cv2 # Open the video file (replace it with your video file path) cap = cv2.VideoCapture('busy-street-in-the-city.mp4') # Get the number of frames per second from the video cap.get(cv2.CAP_PROP_FPS) # Create an instance segmentation object for video segment_video = instance_segmentation(infer_speed="rapid") segment_video.load_model('mask_rcnn_coco.h5') # Process the video and save the output ('frames_per_second' is extracted from the # video using opencv mentioned above which is 30 in our case) result, output = segment_video.process_video( "busy-street-in-the-city.mp4", show_bboxes=True, frames_per_second=30, output_video_name="output_video_street.mp4" )
Click the given link to show the original video without Pixellib effect.
Original Video
The Result Video Link
Make sure to replace the video file path with your own video. This code will segment objects in the video, display bounding boxes, and save the segmented video as "output_video_street.mp4".
That's it! You've now learned how to perform image segmentation using Pixellib, visualize the results, and even extend it to video segmentation. Pixellib simplifies complex computer vision tasks and can be a valuable tool for various applications, including object detection and tracking.
Also Read: Ensemble Models For Flower Image Classification In Deep Learning: A New Era
Real-world Applications of Image Segmentation with PixelLib
Image segmentation itself is a powerful computer vision technique that separates objects in an image into distinct categories. This allows for further analysis and manipulation of those objects. Here are some real-world applications where PixelLib with image segmentation can be a game-changer:
- Background Editing
One of the most popular applications is background removal. PixelLib excels at separating foreground objects from the background. This allows for easy background replacement in photos and videos, perfect for creating special effects or placing objects in new environments.
- Medical Imaging
In the medical field, image segmentation is crucial for analyzing medical scans like X-rays and MRIs. PixelLib can segment tissues, organs, and other structures of interest, aiding in diagnosis and treatment planning.
- Self-Driving Cars
Accurate perception of surroundings is essential for autonomous vehicles. PixelLib with image segmentation helps self-driving cars distinguish between lanes, pedestrians, traffic signs, and other objects on the road to ensure safe navigation.
- Satellite Image Analysis
PixelLib can be used to segment satellite images that allow researchers and analysts to classify land cover, identify objects of interest, and monitor environmental changes.
- Robot Vision
Segmentation is a key function in robot vision systems. PixelLib can help robots identify and grasp objects, navigate their environment, and perform various tasks.
These are just a few examples, and the potential applications of image segmentation with PixelLib are vast. Its ease of use and real-time processing capabilities make it a valuable tool for developers and researchers working on various computer vision projects.
Final Notes
In this blog, we explored the world of image segmentation with PixelLib. We simplify the process and allow you to segment objects in images with just a few lines of code. From installation and model loading to performing segmentation and visualizing results, PixelLib streamlines the workflow.
If you want to learn more about AI and ML, or if you need help implementing AI and ML solutions in your business, CodeTrade India is a great resource. Contact us directly for a free consultation.